How to Get details of your Android device using kotlin
The Android SDK allows application developers to retrieve information i.e device model, device name among other details.
Build Class
import android.os.Build
This class provides a number of static fields and methods which return details of the device. Some of the fields include:
- Build.MODEL: Returns the name of the device.
- Build.VERSION.RELEASE: Returns the current version of the Android operating system
- Build.MANUFACTURER: Returns the name of the manufacturer
- Build.DISPLAY: Returns information about the software version and build type installed on the device.
- Build.FINGERPRINT: Returns a concatenated string containing information about the manufacturer, device model, Android version, and build details.
Android Permissions
It is also possible to get the Wi-Fi/Cellular strength when connected to a network. This would require requesting permissions from the user.
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
<uses-permission android:name="android.permission.READ_PHONE_STATE" />
<uses-permission android:name="android.permission.ACCESS_WIFI_STATE"/>
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION"/>
/* Android requires runtime permission requests starting from Android 6.0 or API level 23 */
fun requestPermissions(fragment: Fragment) {
val requestMultiplePermissionLauncher =
fragment.registerForActivityResult(
ActivityResultContracts.RequestMultiplePermissions()
) { result ->
for (r in result) {
Log.d(Constants.LOG_TAG, r.key + " : " + r.value.toString())
}
}
requestMultiplePermissionLauncher.launch(
arrayOf(
Manifest.permission.ACCESS_COARSE_LOCATION,
Manifest.permission.LOCATION_HARDWARE,
Manifest.permission.ACCESS_FINE_LOCATION,
Manifest.permission.READ_EXTERNAL_STORAGE,
Manifest.permission.WRITE_EXTERNAL_STORAGE,
Manifest.permission.READ_PHONE_STATE,
Manifest.permission.MODIFY_PHONE_STATE,
Manifest.permission.ACCESS_NOTIFICATION_POLICY,
Manifest.permission.BIND_NOTIFICATION_LISTENER_SERVICE,
Manifest.permission.ACCESS_WIFI_STATE,
Manifest.permission.CHANGE_NETWORK_STATE
)
)
}
Code
For simplicity, we have organised the repository in a manner that eliminates most of the boilerplate code.
interface DeviceInfoInterface {
fun getBatteryStatus(): String;
fun getBatteryPercentage(): String
fun getBatteryPercentage_(): Int
fun getTotalStorage(): String;
fun getFreeStorage(): String;
fun getStoragePercentage(): Double;
fun getTotalMemoryUsage(): String;
fun getFreeMemoryUsage(): String;
fun getMemoryPercentage(): Double;
fun getNetworkInfo(): String;
fun getAppVersion(): String;
fun getDataSyncStatus(): String;
fun getDeviceHealth(): String;
fun getUserActivity(): String;
fun getDeviceIdentity(): String;
fun getWifiSignalStrength(): String
fun getCellularSignalStrength(callback: (String) -> Unit);
fun getNetworkType(): String;
fun getUptime(): String;
fun startNetworkCallback(onNetworkChange: (String) -> Unit);
fun stopNetworkCallback();
fun deviceDetails(deviceDetailsBinding: DeviceDetailsBinding);
fun getDeviceId(): String
}
Screens
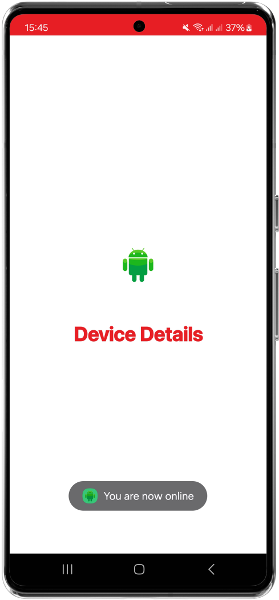
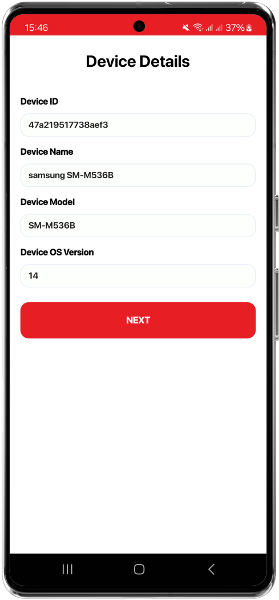
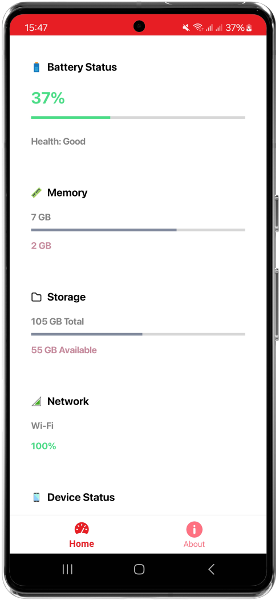
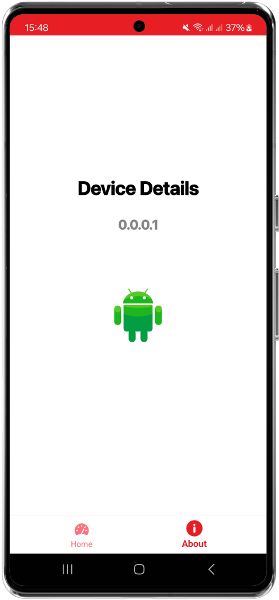
Github
Find the full codebase on Github
Symatech Labs is a Software Development company based in Nairobi, Kenya that specializes in Software Development, Mobile App Development, Web Application Development, Integrations, USSD and Consultancy.